Assignment 5
Due Wed. of Week 7.
Introduction
Throughout history, many resources have been applied to keep information secure or private (e.g., removing the tongues of illiterate servants or slaves). In a computing context, security or cybersecurity is the body of technologies, processes, and practices (which normally do not involve bodily modifications to humans) designed to protect networks, computers, programs and data from attack, damage or unauthorized access.
One method for securing information is encryption. By using a code or cipher, information can be transformed into a pattern that is not understandable, except to those who know how to decode or decrypt the information. There has been an equal amount of energy expended in trying to attain secure information by cracking codes. The computer scientist Alan Turing is famous for his work on cryptanalysis of the German Enigma code during World War II. With the advent of computers, has come the need for cybersecurity and using computers for encryption and decryption.
Implementing simple encryption schemes
You are provided with an abstract base class Cipher
, which has two public methods, encrypt
and decrypt
, which you must implement. Also provided is an example of a ROT13 cipher implementation derived from this class, and a test program that ensures that the encryption and decryption work as expected. You will create more classes that implement different ciphers. Source files: assignment5.tar or assignment5.zip.
Here is the inheritance of the provided interface (Cipher) and one of its subclasses (Rot13Cipher). You must implement the remaining two classes, CaesarCipher and DateCipher and some tests to use them. Example executables are on ix/ix-dev in ~norris/public/cis330/assignment5
.
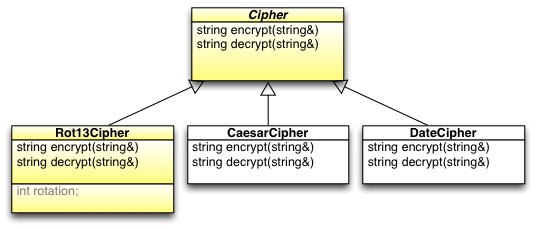
(30 pts) Problem 1. Caesar cipher
Create a class CaesarCipher
that derives from Cipher
and implements the encrypt and decrypt methods for a Caesar cipher. The basic idea in this cipher is that you pick an integer for a key, and shift every letter of your message by the key. For example, if your message was hello and your key was 2, h becomes j, e becomes g, and so on.
In this problem, we will use a variant of the standard Caesar cipher where the space character is included in the shifts: space is treated as the letter after z, so with a key of 2, y would become , z would become a, and would become b.
Modify the Makefile to create caesar.o from your caesar.cpp file.
Required files: caesar.hpp, caesar.cpp, Makefile
(Note: Create a single Makefile with rules for all the problems.)
(10pts) Problem 2. A test program for the Caesar cipher class
Create a test program testCaesar
that uses your new CaesarCipher
class to encrypt and decrypt input in a way similar to the example provided in Rot13Cipher
, but with different logic in the encrypt
and decrypt
methods. Note that a major difference between the ROT13 and other encryption methods is that normally your decrypt
method is not going to be the same as your encrypt
method.
Modify the Makefile to build an executable including your testCaesar.cpp and caesar.cpp files (and any other required files). Make sure the command make testCaeser.exe
works on Linux.
Required files: testCaesar.cpp, Makefile
(30pts) Problem 3. A date shift cipher
Create a class DateCipher
that derives from Cipher
and implements the encrypt and decrypt methods.
- Pick a date. An example would be Steven Spielberg's birthday: December 18, 1946.
- Write out that date using numbers and slash marks: 12/18/46.
- Get rid of the slashes, leaving you with a six-digit number that you will use to encipher your message: 121846. Assume the message is "I enjoy the movies of Steven Spielberg." Under the message, you will write your six digit number over and over until you come to an end: 1 21846 121 846121 84 612184 612184612 is the secret message. However, it is not done yet.
- Write out the alphabet from left to right.
- Shift each letter of the plain text by the number of spaces indicated by the number below it. The letter I shifts one space, making it J; E shifts 2 spaces, making it G. Notice in the word message the Y shifts 6 spaces, causing you to wrap around back to the beginning of the alphabet, landing at E. Your final message would be: "J gorse ujf usbjgt wj Yugwmr Yqkftfksi." (possibly with different capitalization, depends on the alphabet representation you have chosen -- you don't have to match the letter case in the encryption, but it should be decryptable to the original message, including letter case.)
- To implement
decrypt
to decipher this message, simply reverse the process: write out the numerical code, then go back that many spaces of the alphabet.
The Date Shift Cipher has an added advantage into it in that it is fairly random - you can change the date at any time. This allows you to update your system much easier than other ciphers. Famous dates, such as December 7, 1941 (12/07/41), should be avoided, however.
Modify the Makefile to create date.o from your date.cpp file.
Required files: date.hpp, date.cpp, Makefile
(10 pts) Problem 4. A test program for the date cipher
Create a test program testDate
that uses your new DateCipher
class to encrypt and decrypt input in a way similar to the example provided in Rot13Cipher
and the CaesarCipher
you implemented in Problem 1, but with different logic in the encrypt
and decrypt
methods. Do not read in a date from standard input, just hard-code any date you wish in your main
program.
Modify the Makefile to build an executable including your testDate.cpp and date.cpp files (and any other required files). Make sure the command make testDate.exe
works on Linux.
Required files: testDate.cpp, Makefile
(10pts) Makefile for all problems
(10pts) Good Git use practices
Regular commits of incremental changes over the assignment period (the easiest way to get full points is to commit/push any time you need to stop working on the assignment, no matter how briefly). If you only have commits from the due date, you will get 0 points.
Problem 5. Extra credit (Optional, up to 10 extra points)
Create a mini "code breaker" program (testExtra
) that is given an encrypted text as input, decrypts it, and outputs the unencrypted text. You can assume that the input is text encrypted with either ROT13 or Caesar encryption. (Adding date shift decryption makes this much harder because you will not be given the date.)
Add an testExtra:
target to your Makefile so that make extra
builds your implementation of Problem 5 and creates the testExtra
executable which can be used in the same manner as the tests in Problems 2 and 4.